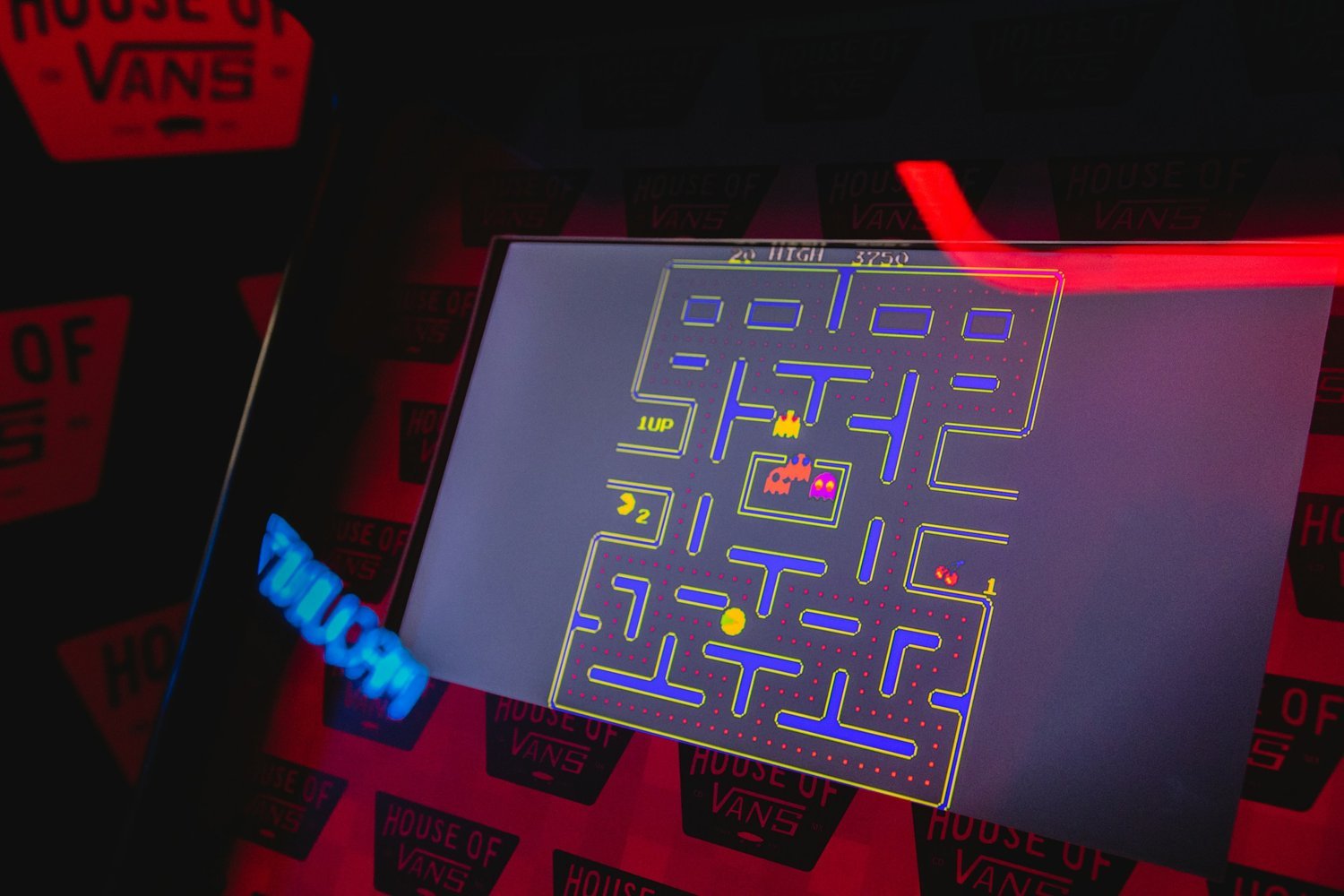
Coding Project - PACMAN in C++
PROJECTS · COMPUTER SCIENCE
Computer Science, Coding Project
300h
March. 2020 - June. 2020
INTRO
Project Overview
This project is a C++ based implementation of a simplified Pac-Man game. The game offers a menu-driven interface that allows players to choose between three modes:
Single-player (vs. computer): The player controls Pac-Man while the computer controls the ghosts.
Two-player mode: Two players control separate Pac-Man characters, competing for the highest score.
Exit game.
If an invalid option is selected, an error message is displayed, and the menu is shown again. The game runs until the user chooses to exit.
SKILLS
Fields of Expertise
Problem Solving & Algorithmic Thinking:
Data Structures & Algorithms:
Software Development (SDLC):
Coding (C++):
Complexity: ★★★☆☆
Tools: C++
Project Type: Uni Project
01
INTRO
CHAPTER
___ INTRO
Game Mechanics
The game board is a 30-row by 20-column grid (600 positions in total). Some positions are navigable, while others serve as obstacles. Movement is turn-based, with Pac-Man and ghosts able to move only to adjacent navigable positions.
Single-player mode: The user controls Pac-Man, while three computer-controlled ghosts attempt to capture it. Ghosts move towards Pac-Man but may move randomly if their direct path is blocked.
Two-player mode: Both players control separate Pac-Man characters, taking turns to move. The player with the highest score at the end wins.
The game ends when Pac-Man visits all navigable positions or gets caught by a ghost (single-player) or when all positions are covered (two-player mode).
Pac-Man gains 100 points for visiting an unvisited position; revisiting positions does not add points.
___ INTRO
Additional Features
File Handling
All in-game messages (score updates, movements, and game state) are logged to a file.
If a predefined board is used, an additional file stores the initial board state.
Global variables are used to manage file operations.
These optional features were also added to the project:
Loading a Predefined Board: The game can load a board configuration from a file, defining navigable spaces, Pac-Man, and ghost positions.
Saving the Game State: Players can save the current board state to a file and reload it later.
Power-Up Mode: Special tiles grant Pac-Man temporary immunity from ghosts. If Pac-Man collides with a ghost during this period, it earns 500 points, and the ghost becomes harmless for the rest of the game.
Board Representation
The board is stored in a 2D array where:
-1
represents an unvisited navigable position (+
on display).0
represents a visited position (*
on display).2
represents a non-navigable position (X
on display).3
represents Pac-Man.8
represents a ghost.At the start, 150 positions are randomly set as non-navigable obstacles.
02
KEY FEATURES
___ FEATURES
Key Functions
The program is modular, with the following key functions:
main()
: Handles file operations, displays the menu, and controls the game loop.menu()
: Displays the menu and validates user input.juego_comecocos(int opcion)
: Starts the game based on the selected mode.jugar_ordenador(int relleno, int comecocos)
: Runs the single-player mode, handling both player and AI movements.jugar_usuario()
: Runs the two-player mode.cargar_tablero_partida(int tablero[FILAS][COLUMNAS])
: Loads a predefined board from a file.guarda_tablero_fichero(int tablero[FILAS][COLUMNAS], char nombreFichero[50])
: Saves the current board state.inicializar_tablero(int tablero[FILAS][COLUMNAS])
: Randomly generates the board if no predefined board is used.movimiento_fantasma(int movimiento[FILAS][COLUMNAS])
: Moves ghosts according to AI logic.movimiento_manual(int tablero[FILAS][COLUMNAS], int jugador)
: Handles player movement input.comprobar_ganador_ordenador(int tablero[FILAS][COLUMNAS])
: Checks victory conditions for single-player mode.comprobar_fin_usuarios(int tablero[FILAS][COLUMNAS])
: Checks victory conditions for two-player mode.
___ FEATURES
Key Challenging Features
Challenge:
Implementing smooth, grid-based movement while allowing responsive input handling is tricky.
Ensuring Pac-Man only moves in valid directions based on the maze layout.
Handling interactions with walls, tunnels, and wrap-around mechanics (e.g., moving from one side of the screen to the other).
Solution Approach:
Use a tile-based movement system, where Pac-Man moves between discrete grid cells.
Maintain a queue for directional inputs, ensuring Pac-Man turns at the nearest available opportunity rather than instantly.
Implement collision detection to check whether the next intended move is valid before executing it.
Allow for smooth movement by interpolating Pac-Man’s position between grid cells, making movement feel fluid rather than rigid.
___ KEY FEATURE 1
Pac-Man Movement and Collision Handling
__ KEY FEATURE 1
Pac-Man Movement and Collision Handling
__ KEY FEATURE 2
AI for Ghost Behavior (Pathfinding & State Management)
. . .
__ KEY FEATURE 3
Power Pellet Mechanics (Pac-Man Eating Ghosts)
Challenge:
Ghosts exhibit different behaviors based on their AI personalities (e.g., Blinky chases Pac-Man, Pinky predicts his movement, Inky uses Blinky’s position for strategy, and Clyde moves randomly at times).
Implementing pathfinding so ghosts efficiently navigate the maze rather than moving randomly.
Managing different states: chase, scatter, and frightened, with smooth transitions.
Solution Approach:
Implement BFS (Breadth-First Search)* for pathfinding, allowing ghosts to find optimal paths towards Pac-Man.
Use a finite-state machine (FSM) to control each ghost’s state, transitioning between:
Chase Mode: Actively pursuing Pac-Man using pathfinding.
Scatter Mode: Moving to predefined corners of the map.
Frightened Mode: Moving randomly if Pac-Man eats power pellet or if colliding with an obstacle.
____ KEY FEATURE 2
AI for Ghost Behavior (Pathfinding & State Management)
Challenge:
Temporarily changing game rules when Pac-Man eats a power pellet.
Making ghosts turn blue and move erratically.
Allowing Pac-Man to eat ghosts, sending them back to their starting position.
Resetting ghost behavior when the effect wears off.
Solution Approach:
Upon eating a power pellet, trigger a state change in all ghosts to “frightened” mode.
Modify ghost AI to move randomly instead of using pathfinding.
If Pac-Man collides with a ghost in this state:
The ghost disappears momentarily.
It respawns at its home position after a delay.
Implement a countdown timer to revert ghosts back to their normal behavior after a few seconds.
____ KEY FEATURE 3
Power Pellet Mechanics (Pac-Man Eating Ghosts)
SEE MORE PROJECTS
MATH, PHYSICS & ENGINEERING PROJECTS
COMPUTER SCIENCE PROJECTS
ML & AI PROJECTS
QUANT FINANCE PROJECTS