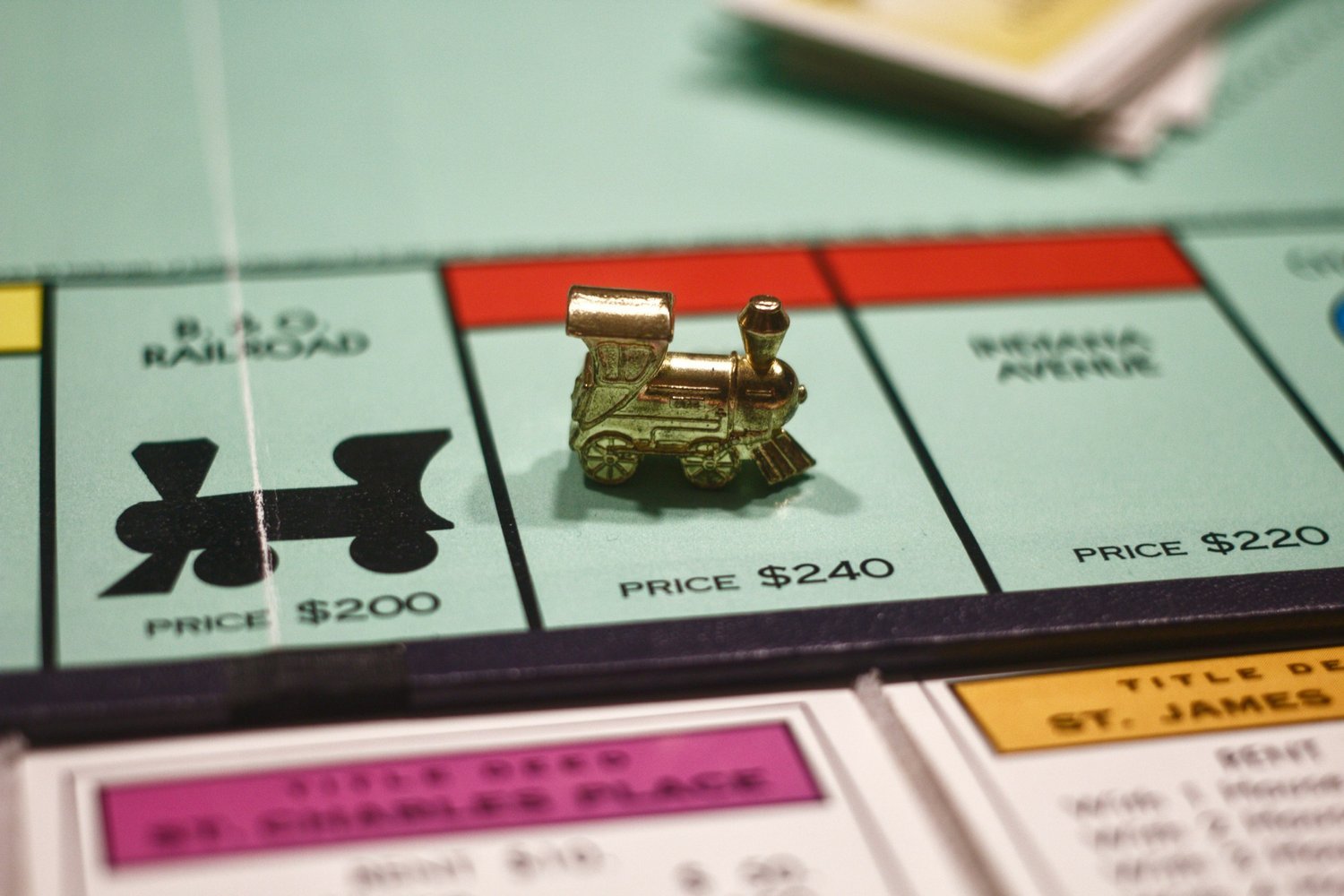
Coding Project - Monopoly in JAVA
PROJECTS · COMPUTER SCIENCE
Computer Science, Coding Project
300h
March. 2020 - June. 2020
INTRO
Project Overview
The aim of this project is the development of a Monopoly game simulation in Java, employing Object-Oriented Programming (OOP) principles to model game entities and interactions systematically. Core components, such as players, properties, and the game board, were abstracted into discrete classes, utilizing encapsulation, inheritance, and polymorphism to ensure modularity and extensibility.
The implementation focused on separating concerns, with each class responsible for its state and behavior, while maintaining clear interfaces for interaction. This approach facilitated the exploration of OOP design patterns, algorithmic logic for game mechanics, and robust error handling, resulting in a structured and scalable simulation of the Monopoly game system.
SKILLS
Fields of Expertise
Problem Solving & Algorithmic Thinking:
Object Oriented Programming (OOP):
Software Development (SDLC):
Coding (JAVA):
Complexity: ★★★★☆
Tools: JAVA
Project Type: Uni Project
01
INTRO
CHAPTER
___ INTRO
Project Description
The program aims to simulate the popular board game "Monopoly". The main interface of the program is the command line, which displays all the information relative to the game, and allows us to navigate through the menus and introduce information. In addition, an extra Java Panel has been added to the interface of the command line, which will allow the players to throw the dices each turn, and will show the information relative to the players at all times (name, dices, money and position in the board), in order to make it simple for the users to quickly know the situation of the game at a glance.
___ STEP 1
Program Initialization
At the initialization of the program, the user is asked to introduce the number of players (which is limited from 2 to 6), and the names and colors of each player. Obviously, two players are not allowed to have the same name or color. After the initialization of the program, each player shall throw the dices in order to define the order of the game. At this point, the new panel window will open, and will show from now on all the information of the players at all times. Is very important to clarify here that each player has his own button to roll the dices, which must be pushed only in the corresponding turn of that player, and only once.
___ STEP 3
___ STEP 2
Player Turns
After rolling the dices for each player and pressing INTRO, the orders of the turns are defined, and the game is ready to start! The turns of the players are always structured in the same way. The corresponding player rolls the dices, and the program automatically displays the number of the square where the player has moved, and the specific information about the square, depending on the class. If it is a Title Deed (property, station or utility/company), information about the owner, the purchase price, and the rent is displayed. In case the square is a card or a special square, the information is also displayed on screen.
Main Menu
After the corresponding information has been displayed, the main options menu for each turn is shown. The menu offers all the available possibilities for the players. In case they are in a title deed square, the BUY option is first displayed. If they want to see their properties first before buying, they can access the manage properties option, which automatically displays all the title deeds that the player has in possession. After selecting any of them, a new menu emerges, this time showing additional options on building or selling houses and hotels, or going back to the main options menu. Moreover, it is possible to access to further actions, encompassed under the "trade property" option. This will allow the player to sell the selected property to any other player, or to buy other properties belonging to any other player.
___ STEP 4
Buying Properties
After exploring all the options of the menu, the player can decide whether or not to buy the property landed. In case he doesn’t want to, he can select: "end turn". In this case, the auction for the property automatically starts, and the player who has rejected the purchase can still participate. All the players can submit their bids, and the player with the higher bid at the end of the round is the one who will purchase the property. If all the players introduce the number 0, the property will not be purchased by anyone.
02
KEY FEATURES
___ FEATURES
UML Diagram
___ FEATURES
Key Challenging Features
Challenge:
The Monopoly board consists of 40 distinct spaces, each with unique behaviors (properties, utilities, railroads, chance/community chest cards, taxes, jail, etc.). Hardcoding these behaviors would make the system rigid and difficult to extend or modify.
Solution Approach:
Implemented an Object-Oriented approach with an abstract
BoardSpace
class and specific subclasses (Property
,ChanceCard
,TaxSpace
, etc.), allowing each type of space to define its own behavior.Used a Factory Pattern to dynamically create board spaces based on configuration files or JSON data, ensuring easy modifications.
Stored board spaces in an ArrayList, enabling efficient traversal and easy modifications in case of rule variations.
___ KEY FEATURE 1
Implementing Flexible & Scalable Game Board
__ KEY FEATURE 1
Implementing Flexible & Scalable Game Board
__ KEY FEATURE 2
Managing Complex Player Interactions & Game Logic
. . .
__ KEY FEATURE 3
Implementing Property Auctions
Challenge:
Players must interact with various game elements such as buying/selling properties, paying rent, drawing chance/community chest cards, and handling in-game transactions. Ensuring turn-based gameplay while managing all edge cases (bankruptcy, jail rules, house/hotel purchases, etc.) added significant complexity.
Solution Approach:
Implemented a State Machine to track player actions within their turn (
ROLL_DICE
,BUY_PROPERTY
,PAY_RENT
,DRAW_CARD
, etc.), ensuring a structured sequence of events.Used an Event-Driven Architecture, where player actions triggered specific game events (e.g.,
LandOnPropertyEvent
,BankruptcyEvent
), making interactions modular and easier to debug.Maintained a transaction system that handled cash flow between players and the bank, ensuring correct balance updates and enforcing rules like mortgage & auction handling.
____ KEY FEATURE 2
Managing Complex Player Interactions and Game Logic
Challenge:
When a player declines to buy a property, Monopoly rules state that the property goes up for auction, where all other players can bid. Simulating a fair, dynamic auction system where multiple players (human and AI) can place bids while ensuring smooth game flow was challenging.
Solution Approach:
Created an AuctionManager class that handled bidding rounds, keeping track of the highest bid and active participants.
Implemented a bidding strategy for AI players, where their decisions were based on:
Available cash balance.
Property’s strategic value (e.g., completing a color set).
Opponent holdings (avoiding overpaying if an opponent is close to bankruptcy).
Designed a turn-based auction loop, where players sequentially placed bids or withdrew until only one remained. This ensured a fair and interactive bidding process.
Allowed human players to input their bid amounts dynamically, ensuring a competitive auction experience.
____ KEY FEATURE 3
Implementing Property Auctions
SEE MORE PROJECTS
MATH, PHYSICS & ENGINEERING PROJECTS
COMPUTER SCIENCE PROJECTS
ML & AI PROJECTS
QUANT FINANCE PROJECTS